Authentication
All API calls require both a Key and ID.
Restricting Access
In order to make any API call you must pass the API Key & ID with every request. The key/ID can be passed in either the header, body or as a query parameter.
- Header: When sending the authentication tokens in the header they would have the format 'api-id' and 'api-key'.
- Params/Body: When sending the authentication tokens in the body or are parameters, they would be in the format 'api_id' and 'api_key'.
Authentication Validation API
We have a validation API that can be used to validate your credentials.
/api/v1/authenticate (both POST and GET requests)
curl https://api.retention.com/api/v1/validate?api_key=API_KEY&api_id=API_ID
resp, err := http.Get("https://api.retention.com/api/v1/validate?api_key=API_KEY&api_id=API_ID")
if err != nil {
// handle err
}
defer resp.Body.Close()
import requests
params = {
'api_key': 'API_KEY',
'api_id': 'API_ID'
}
response = requests.get('https://api.retention.com/api/v1/validate', params=params)
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.retention.com/api/v1/validate?api_key=API_KEY&api_id=API_ID');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
$result = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
}
curl_close($ch);
require 'net/http'
require 'uri'
uri = URI.parse("https://api.getemails.com/api/v1/validate?api_key=API_KEY&api_id=API_ID")
response = Net::HTTP.get_response(uri)
# response.code
# response.body
import java.io.IOException;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Scanner;
class Main {
public static void main(String[] args) throws IOException {
URL url = new URL("https://api.retention.com/api/v1/validate?api_key=API_KEY&api_id=API_ID");
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
httpConn.setRequestMethod("GET");
InputStream responseStream = httpConn.getResponseCode() / 100 == 2
? httpConn.getInputStream()
: httpConn.getErrorStream();
Scanner s = new Scanner(responseStream).useDelimiter("\\A");
String response = s.hasNext() ? s.next() : "";
System.out.println(response);
}
}
Where to get your API Credentials
You can create your API Key and ID from your Retention.com dashboard. Please follow the steps below:
- Go to your Retention.com dashboard
- From the navigation bar on the left, click on "My Account"
- Select "API Details" from the options
- Click on "Create New Credentials" at the top of the screen
- Give an appropriate name and description for the credentials, then click "Create"
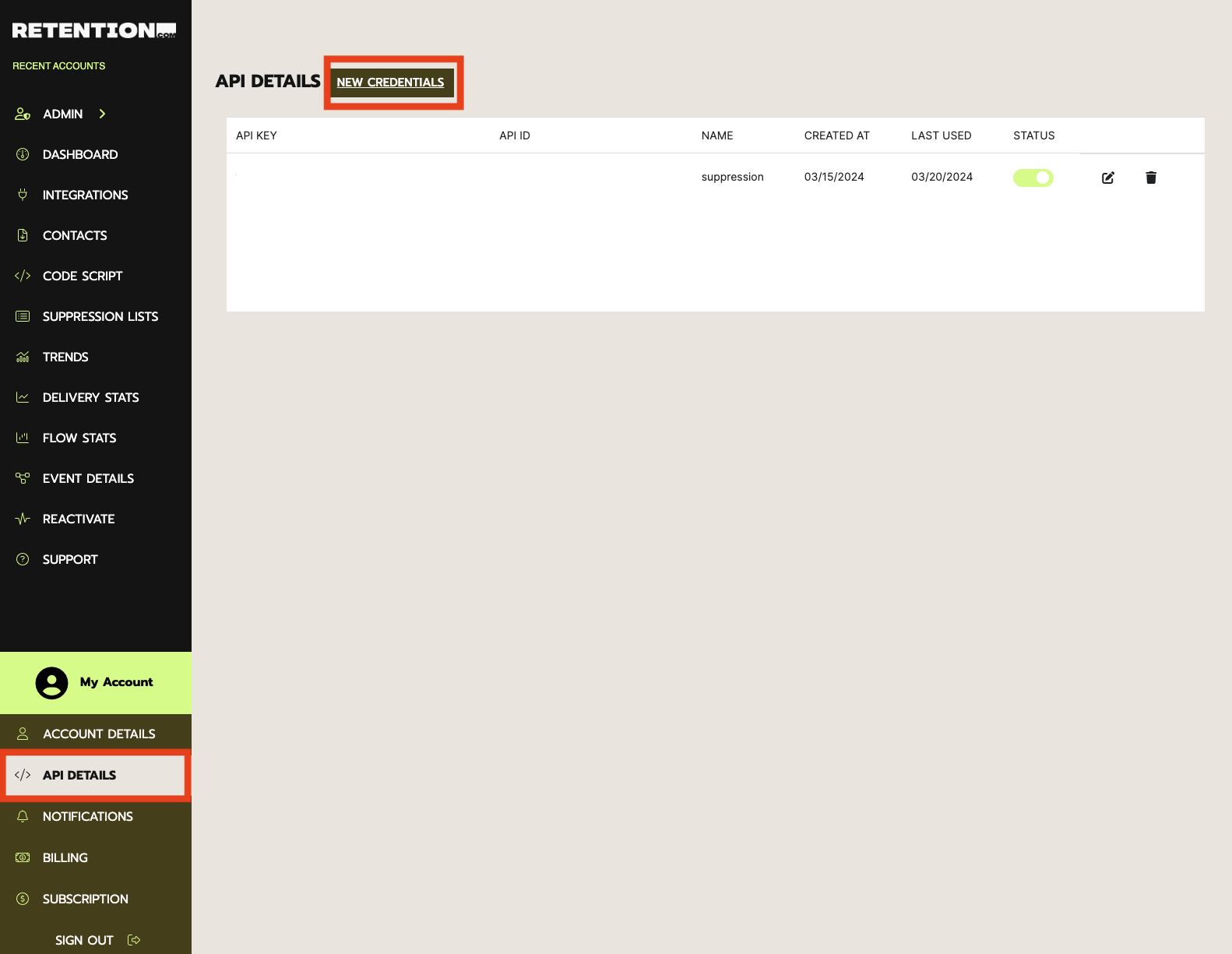
Updated 9 days ago